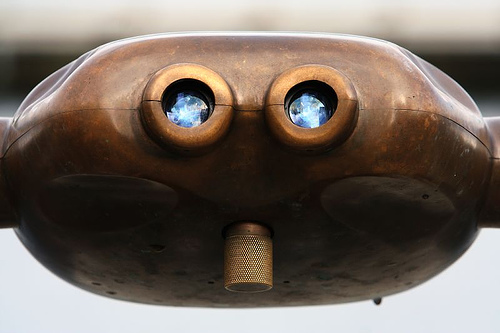
“Just what the world needs—another sucky JavaScript library,” he said. When I asked him what made it ‘sucky’, he elaborated. “It’s a JavaScript library written by Java developers who clearly don’t get JavaScript.”
This quote is by Kevin Yank, talking to Dmitry Baranovskiy, author of the fantastic Raphaël graphics abstraction library. He writes up more on the issues they see with Closure. This is the few of one side of the community towards the open source release of Google Closure and its tools.
It was bound to happen. When I started at Google I quickly wanted to learn about how Gmail, Google Maps, and all the revolutionary front ends that helped make Ajax happen. Closure was the heart of it, and I quickly wanted to see a way to get the knowledge out there. This was years ago now, but the same reasons permeate through. Even at the time there were a ton of great JavaScript libraries. It wasn’t that I wanted Google to come down the mountain like Moses and bless the world “thou shalt use Closure!” However, some smart people had worked for some time refining their use of the browser runtime and Closure was the art and science of that ongoing experiment. Having the world able to at least see the experiment would be a good thing. Other libraries could learn from it.
No one from Google has said that you should, or must use Closure!
I realize that we live in a world of heros or zeroes. There isn’t room for much in between. You are either going to be a X killer, or you are killed by X. There is no time to tell the longer arching tale. Closure came to us late in the game, where folks are often perceived to be in their camps. I love JavaScript because it allows a diverse group of libraries to do what they do best. Developers and designers can find what suits both their personalities and their particular project.
If someone asks me (and they often do) “Which Ajax library should I use?” there isn’t a simple answer. It is nuanced. It is complicated. It is frustrating! How many of you have even changed libraries in a project? It is embarrassing to go back in time and see what happened with Bespin. At various times we had MooTools, Prototype, Dojo, and SproutCore. Is that productive? Obviously no.
You build many things on the Web. A web site isn’t a web application, and then blend together so you often can’t tell when you go from one to the other (and it doesn’t of course matter at all). jQuery has made a living starting out as a fantastic DSL for sprinkling behaviour onto web sites and going from there. SproutCore and Cappuccino are the other extreme, and Dojo was the kitchen sink of JS development a long time ago. Speaking of Dojo, I really wish that Dojo and Closure had collided a long time ago. I think that it would have benefitted both sides, even though I know that it would have added some overhead for both too. When you are on an internal framework it is built for your needs. If you take a look at the Closure components they feel very Googley out of the box. At the end of the day though, Dojo and Closure are so similar in many ways, that having them joint at the hip a few years back would have been really exciting. C’est la vie.
One tricky thing about Closure is that you can’t look at all of the widgets and packages equally (same for most libraries to be fair). Some are battled hardened to the hilt as they are on important production systems acting as core functionality. The keyboard handling and localization support is top draw. Others may be cool but placed in via an interesting 20% project that isn’t as mission critical and hence battle hardened. Not all code is equal. In fact, I find the line by line code criticism tiring. It is so easy to take someones code and find fault with it (at least perceived fault). I am sure there is bad code in Closure. That is true for all code. It is much harder to be a creator though. Don’t get me wrong, it is good to point out potential issues, but there is a big difference between:
“I noticed that code X does Y. I think there is a bug there that would affect ….”
and
“Code X SUCKS. Mwhahahahahah. SUCKS!”
My new years resolution for 2010: “Say *sucks* less”.
The criticism that made me laugh hardest was the assertion that the Closure engineers were Java folks who wanted JS to be Java. They are far from it. They are old school JS engineers who have done amazing things on the Web and have just learned a few things along the way as they build huge scale JS systems.
Google is of course a big place with engineers with opinions :) When you have the creators of various languages and platforms under one roof that is always going to be the case (From Python to C++ to Java to Go, and then Closure, GWT, etc on the JS side… even jQuery ;). Many of the frontend engineers aren’t fans of Closure (as in: doesn’t fit them) but they do understand where some of the strengths are. Some of the engineers have even weighed in:
NOTE: The following quotes were taken from a Facebook comment stream. There is context missing (for example, Joel talks below about how he didn’t use the term ‘natives’ for example
Joel Webber, GWT guru: “I’m unfortunately not too surprised to see self-appointed “Javascript natives” pissing all over Closure, because it tells them something they don’t want to hear — namely, that you can’t get enough optimization leverage on large applications without some constraints on your code’s structure. The kind of stuff that you see in many Javascript libraries (e.g. lots of dynamic tests to figure out what to do in a function) pretty much guarantees that you’ll have an unoptimizeable mess.”
Gavin Doughtie, Dojo and Google Photos: “As one of the Javascript natives, I must say after three years of using Closure that it gives you a nice split between development-time dynamism and deployment-time optimization. I have found few Javscript idioms unavailable to me when coding, yet still benefit from static checking and optimizations once I’m ready to ship.”
I am more of a JavaScript native than anything else. I am not going to be rushing to use Closure on a project any time soon. It doesn’t fit many of the projects I work on to be honest, and it doesn’t fit me as well as some of the other libraries.
That being said, I am thankful to Google for putting this code in the commons (and the fantastic tools around it!) so we can all check it out (and some of us use it). I hope that the hard part of open sourcing a project kicks in and we see a community form around it, including taking in contributions. I still also harbor the hope that Dojo and Closure joined forces, but that is hard to do (a lot of people using both code bases!)
When it comes to getting closure, I hope that you don’t jump to the extreme and ignore and abuse it, and I also hope that you also don’t think that you SHOULD use it without checking it out and understanding the tradeoffs.
For example, this is crazy:
“I hate to admit but I “might” have switched from jQuery to Closure strictly because of the Google brand.”
Roundup on Closure